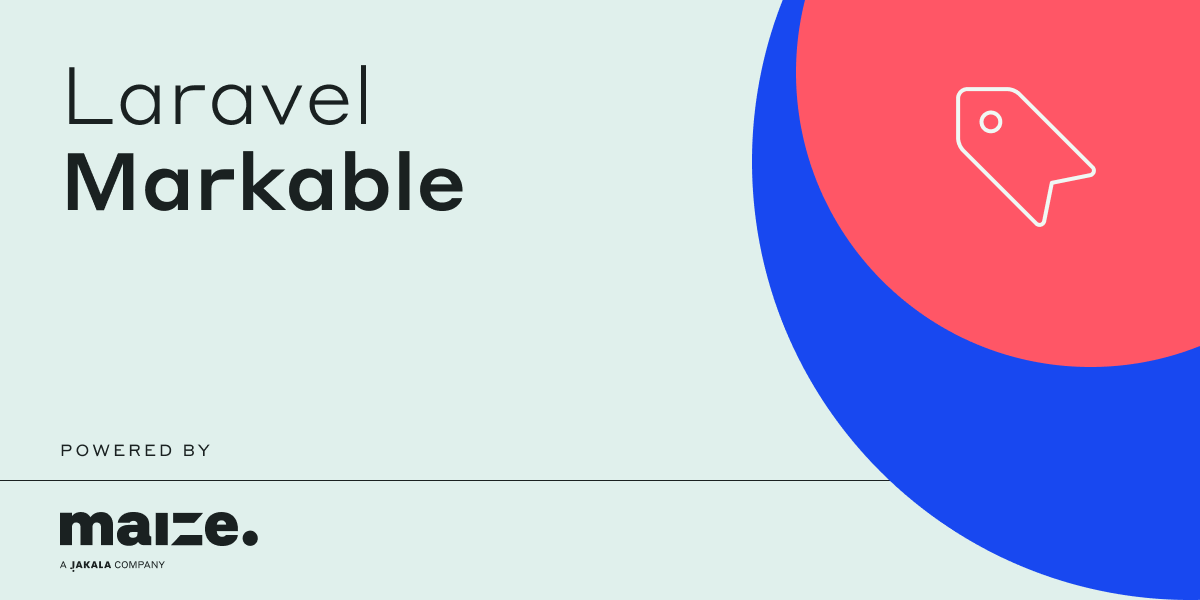
https://github.com/maize-tech/laravel-markable/tree/main
安装
你可以通过 composer 安装这个包:
composer require maize-tech/laravel-markable
你可以发布并运行迁移文件:
php artisan vendor:publish --tag="markable-migration-bookmark" # 发布书签迁移
php artisan vendor:publish --tag="markable-migration-favorite" # 发布收藏迁移
php artisan vendor:publish --tag="markable-migration-like" # 发布点赞迁移
php artisan vendor:publish --tag="markable-migration-reaction" # 发布反应迁移
php artisan migrate
你可以发布配置文件:
php artisan vendor:publish --tag="markable-config"
这是发布的配置文件的内容:
<?php
return [
/*
|--------------------------------------------------------------------------
| User model
|--------------------------------------------------------------------------
|
| Here you may specify the fully qualified class name of the user model class.
|
*/
'user_model' => App\Models\User::class,
/*
|--------------------------------------------------------------------------
| Table prefix
|--------------------------------------------------------------------------
|
| Here you may specify the prefix for all mark tables.
| If set, all migrations should be named with the given prefix and
| the mark's class name.
|
*/
'table_prefix' => 'markable_',
/*
|--------------------------------------------------------------------------
| Allowed values
|--------------------------------------------------------------------------
|
| Here you may specify the list of allowed values for each mark type.
| If a specific mark should not accept any values, you can avoid adding it
| to the list.
| The array key name should match the mark's class name in lower case.
|
*/
'allowed_values' => [
'reaction' => [],
],
];
使用
基础
要使用这个包,将 Maize\\Markable\\Markable
trait 添加到你想要有标记功能的模型中。
一旦完成,你可以定义给定模型的可能标记列表,通过实现 $marks
数组,并列出标记类的命名空间。
这里有一个包括 Markable
trait 和实现 Like
标记的模型示例:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Maize\Markable\Markable;
use Maize\Markable\Models\Like;
class Course extends Model
{
use Markable;
protected $fillable = [
'title',
'description',
];
protected static $marks = [
Like::class,
];
}
你现在可以给模型分配点赞:
use App\Models\Course;
use Maize\Markable\Models\Like;
$course = Course::firstOrFail();
$user = auth()->user();
Like::add($course, $user); // marks the course liked for the given user
Like::remove($course, $user); // unmarks the course liked for the given user
Like::toggle($course, $user); // toggles the course like for the given user
Like::has($course, $user); // returns whether the given user has marked as liked the course or not
Like::count($course); // returns the amount of like marks for the given course
自定义元数据
如果需要,你还可以在分配标记时添加自定义元数据:
use App\Models\Course;
use Maize\Markable\Models\Like;
$course = Course::firstOrFail();
$user = auth()->user();
Like::add($course, $user, [
'topic' => $course->topic,
]);
Like::toggle($course, $user, [
'topic' => $course->topic,
]);
自定义标记模型
这个包允许你定义自定义标记。
首先,你需要创建一个定义新标记模型的迁移。该包使用单独的表格为每个标记提供性能优化。
迁移表的名称应包含在 config/markable.php
下的 table_prefix
属性中定义的前缀。默认前缀设置为 markable_
。
这里有一个书签迁移的示例:
return new class extends Migration
{
public function up()
{
Schema::create('markable_bookmarks', function (Blueprint $table) {
$table->id();
$table->foreignId('user_id')->constrained()->cascadeOnUpdate()->cascadeOnDelete();
$table->morphs('markable');
$table->string('value')->nullable();
$table->json('metadata')->nullable();
$table->timestamps();
});
}
}
完成后,你可以创建一个新的类,该类扩展了抽象的 Mark
类,并实现了 markableRelationName
方法,该方法用于检索标记了给定模型实体的用户,以标记实体为枢纽。
你还可以覆盖 markRelationName
方法,该方法用于检索给定模型实体的标记列表。默认情况下,关系名称是标记类名称的复数形式。
这里有一个书签标记模型的示例:
<?php
namespace App\Models;
use Maize\Markable\Mark;
class Bookmark extends Mark
{
public static function markableRelationName(): string
{
return 'bookmarkers';
}
/**
* The override is useless in this case, as I am returning the default
* relation name which is the plural name of the mark class name (bookmarks, indeed)
*/
public static function markRelationName(): string
{
return 'bookmarks';
}
}
这样就完成了!你现在可以将自定义标记包含到所有你希望的模型中,并像之前解释的那样使用它。
使用标记值
你可能需要一个具有一组允许值的自定义标记。
在这种情况下,你可以像之前解释的那样定义你的自定义标记,并在 config/markable.php
下的 allowed_values
数组中添加允许值的列表。
数组的键名应与标记的类名(小写)匹配。
这里有一个与反应相关的示例:
'allowed_values' => [
'reaction' => [
'person_raising_hand',
'heart',
'kissing_heart',
],
],
你可以使用带有值的自定义标记:
use App\Models\Post;
use Maize\Markable\Models\Reaction;
$post = Post::firstOrFail();
$user = auth()->user();
Reaction::add($post, $user, 'kissing_heart'); // adds the 'kissing_heart' reaction to the post for the given user
Reaction::remove($post, $user, 'kissing_heart'); // removes the 'kissing_heart' reaction to the post for the given user
Reaction::toggle($post, $user, 'heart'); // toggles the 'heart' reaction to the post for the given user
Reaction::has($post, $user, 'heart'); // returns whether the user has reacted with the 'heart' reaction to the given post or not
Reaction::count($post, 'person_raising_hand'); // returns the amount of 'person_raising_hand' reactions for the given post
你还可以使用通配符允许特定标记的任何值。
这里有一个与反应相关的示例:
'allowed_values' => [
'reaction' => '*',
],
当设置后,你可以使用任何值进行反应:
use App\Models\Post;
use Maize\Markable\Models\Reaction;
$post = Post::firstOrFail();
$user = auth()->user();
Reaction::add($post, $user, 'random_value'); // adds the 'random_value' reaction to the post for the given user
使用 Eloquent 检索实体的标记列表
use App\Models\Course;
use App\Models\Post;
Course::firstOrFail()->likes; // returns the collection of like marks related to the course
Post::firstOrFail()->reactions; // returns the collection of reaction marks related to the post
使用 Eloquent 检索标记了实体的用户列表
use App\Models\Course;
use App\Models\Post;
Course::firstOrFail()->likers; // returns the collection of users who liked the course along with the mark value as pivot
Post::firstOrFail()->reacters; // returns the collection of users who reacted to the post along with the mark value as pivot
使用 Eloquent 过滤标记了模型的用户
use App\Models\Course;
use App\Models\Post;
Course::whereHasLike(
auth()->user()
)->get(); // returns all course models with a like from the given user
Post::whereHasReaction(
auth()->user(),
'heart'
)->get(); // returns all post models with a 'heart' reaction from the given user
测试
composer test
更新日志
请查看 CHANGELOG 以获取最近更改的更多信息。
贡献
请查看 CONTRIBUTING 了解详情。
安全漏洞
请查看 我们的安全政策 了解如何报告安全漏洞。
致谢
许可证
MIT 许可证。请查看 许可证文件 了解更多信息。